namespace
Graphics
Contents
- Reference
Namespaces
- namespace priv
Classes
- class Atlas
- Generic 2D atlas class.
- struct Batch2DVertex
-
template<typename V>class BatchRenderer2D
-
template<typename T, unsigned int N = 1>class Bitmap
- A 2D bitmap image with N channels of elements T.
-
template<typename T, unsigned int N = 1>class BitmapAtlas
- A 2D bitmap atlas.
- class BRDFLUT
- class Buffer
- struct CameraBuffer
- class ColorModule
- class CommandBuffer
- class D3DBuffer
-
template<class CB>class D3DConstantBuffer
- class D3DDepthTexture
- class D3DFrameBuffer
- class D3DIndexBuffer
- class D3DMappedResource
- class D3DMesh
- struct D3DRendererCache
- class D3DRenderTexture
- class D3DResource
- class D3DShader
- class D3DStates
- class D3DTexture
- class D3DVertexBuffer
- class DeferredRenderer
- class DirectionalLight
- class EmissionModule
- Affects the rate of particle creation.
- class EnviromentMap
- class Font
- Represents a single typeface.
- class FontCollection
- A collection of Glyphs and metadata.
- class ForwardRenderer
- class FrameBuffer
-
template<class T>class GenericLight
- class GLBuffer
- class GLFrameBuffer
- class GLIndexBuffer
- class GLMesh
- class GLShader
- class GLStates
- class GLTexture
-
template<class T>class GLUniformBuffer
- class GLVertexArrays
- class GLVertexBuffer
- struct GlyphMetrics
- Single glyph metrics.
- class ImGuiRenderer
- class Light
- class LookUpTexture
- class ManagedShader
- class MappedResource
- class Material
- struct MaterialUniforms
- class Mesh
- struct MeshEntry
- class MeshGenerator
- struct MMatrix
- class Model
- struct ObjectBuffer
- struct PaintStyle
- struct Particle
- class ParticleEmitter
- class ParticleModule
- Base particle module.
- class ParticleSystem
- struct Path
- struct PathPoint
- class PathRenderer
- class PathRenderer2D
- struct PathRenderer2DVertex
- struct PathRendererCall
- struct PathRendererUniformData
- class Pipeline
- struct PMatrix
- class PointLight
- struct PVMatrices
- struct RenderCommand
- class Renderer
- class Renderer2D
- class Renderer3D
- class Shader
- struct ShaderAttribute
- struct ShaderBuffer
- struct ShaderCompilationResult
- class ShaderCompiler
- class ShaderLoader
- class ShaderPass
- struct ShaderReflection
- struct ShaderResource
- struct ShaderUniform
- class ShapeModule
- Defines the shape from which the particles can be emitted, and their initial direction and speed.
- class SizeModule
- class Skybox
- class SpriteBatcher
- class SpriteRenderer
- class States
- class SurfaceShader
- class TextRenderer2D
- struct TextRenderer2DVertex
- struct TextStyle
- class Texture
- class TextureArrayHandler
- class TextureAtlas
- A 2D texture atlas.
- struct TextureFormatProperties
- class TextureModule
- struct TextureProperties
- struct TextureSampleProperties
-
template<class T>class UniformsPack
- struct Vertex3D
- class VertexBuffer
- struct VertexElement
- class VertexLayout
- class VKFrameBuffer
- class VKPipeline
- class VKShader
- struct VMatrix
Enums
- enum SimulationSpace { LOCAL, WORLD }
- enum PathRendererCommandType { MOVETO = 0, LINETO, BEZIERTO, CLOSE }
- enum MaterialMapType { ALBEDO = 0, METALLIC, ROUGHNESS, NORMALS }
- enum BufferType { VERTEX = 0, INDEX, CONSTANT }
- enum BufferMode { DEFAULT = 0, DYNAMIC }
- enum BlitOperation { DEPTH }
- enum MappedPointerMode { READ, WRITE }
- enum Topology { POINTS, TRIANGLES, TRIANGLES_STRIP, TRIANGLES_FAN, QUADS, PATCHES }
- enum ShaderType { UNKNOWN = 0, VERTEX, VERTEX = 0, FRAGMENT, GEOMETRY, TESS_CONTROL, TESS_EVAL }
- enum ShaderUniformType { UNKNOWN, BOOL, INT, UINT, HALF, FLOAT, DOUBLE }
- enum BlendState { SRC_ALPHA = 0, ONE_ALPHA, NO_COLOR, ONE_ONE, ONE_ZERO, DISABLED }
- enum CullMode { FRONT = 0, BACK, WIREFRAME, NONE }
- enum FunctionMode { NEVER = 0, LESS, EQUAL, LESS_EQUAL, GREATER, NOT_EQUAL, GREATER_EQUAL, ALWAYS, DISABLED }
- enum StencilOperation { ZERO = 0, KEEP, SEPARATE_INCR_DECR }
- enum TextureFormat { R8 = 0, RGB, R16, RG8, RG32F, RGB8, RGB32F, RGB16F, RGBA, RGBA8, RGBA32F, SRC_ALPHA, SRC_ALPHA = 0, LUMINANCE_ALPHA, DEPTH, DEPTH, DEPTH_STENCIL }
- enum TextureFilter { ANISOTROPIC = 0, LINEAR, NEAREST }
- enum TextureWrap { REPEAT = 0, MIRROR_REPEAT, CLAMP, CLAMP_TO_EDGE, CLAMP_TO_BORDER }
- enum TextureDataType { UNSIGNED_INT, UNSIGNED_BYTE, FLOAT, FLOAT }
- enum TextureDimension { TEXTURE1D, TEXTURE2D, TEXTURE2DARRAY, TEXTURE3D, CUBEMAP }
- enum GlyphRenderMode { GRAY = 0, SDF = 1, PSDF = 2, MSDF = 3, COLOR = 4 }
- enum SDFMode { SDF, PSDF, MSDF }
- msdfgen's modes
Typedefs
- using ShaderSources = std::map<ShaderType, std::string>
- using ShaderReflections = std::map<ShaderType, ShaderReflection>
- using ShaderDefinitions = std::set<std::string>
- using FrameIndex = unsigned int
- Frame identifier within an atlas. It must be unique and does not have to be sequential.
- using BitmapRGB = Bitmap<unsigned char, 3>
- using BitmapRGBA = Bitmap<unsigned char, 4>
- using BitmapAtlasRGB = BitmapAtlas<unsigned char, 3>
- using BitmapAtlasRGBA = BitmapAtlas<unsigned char, 4>
- using GlyphCodepoint = unsigned int
- Unicode codepoint.
- using FontSize = unsigned int
- Font sizes specified in pixels.
Functions
-
template<typename T, unsigned int N, typename U, unsigned int M>auto ConvertBitmap(const Bitmap<T, N>& input) -> Bitmap<U, M>
- Convert between bitmaps.
-
template<>auto ConvertBitmap(const Bitmap<unsigned char, 1>& input) -> BitmapRGB
- Convert from unsigned char 8bit (grayscale) to unsigned char 24bit (RGB)
-
template<>auto ConvertBitmap(const Bitmap<unsigned char, 1>& input) -> BitmapRGBA
- Convert from unsigned char 8bit (grayscale) to unsigned char 32bit (RGBA)
-
template<>auto ConvertBitmap(const Bitmap<unsigned char, 3>& input) -> BitmapRGBA
- Convert from unsigned char 24bit (RGB) to unsigned char 32bit (RGBA)
-
template<>auto ConvertBitmap(const Bitmap<unsigned char, 4>& input) -> BitmapRGB
- Convert from unsigned char 32bit (RGBA) to unsigned char 24bit (RGB) (drop alpha)
-
template<>auto ConvertBitmap(const Bitmap<float, 1>& input) -> BitmapRGB
- Convert from float 8bit (grayscale) to unsigned char 24bit (RGB)
-
template<>auto ConvertBitmap(const Bitmap<float, 3>& input) -> BitmapRGB
- Convert from float 24bit (RGB) to unsigned char 24bit (RGB)
- auto ConvertBGRAtoRGBA(const Bitmap<unsigned char, 4>& input) -> BitmapRGBA
- Convert a RGBA bitmap from BGRA to RGBA.
- auto ConvertAlphaToWhiteRGBA(const Bitmap<unsigned char, 1>& input) -> BitmapRGBA
- auto ReadImage(const std::string& path, unsigned int& width, unsigned int& height, unsigned int& bpp, bool force32bits = false) -> unsigned char*
- Reads an image from disk.
- auto ReadImage(unsigned char* file_data, size_t file_size, unsigned int& width, unsigned int& height, unsigned int& bpp, bool force32bits = false) -> unsigned char*
- Reads an image from disk.
- auto WritePNG(const std::string& path, unsigned int width, unsigned int height, unsigned int bpp, unsigned char* data) -> bool
- Writes a PNG file to disk.
-
auto GenerateBitmapFromOutline(FT_
Outline* outline, SDFMode mode, double range) -> BitmapRGB - Generates a BitmapRGB from a FreeType outline using msdfgen's signed distance field method.
-
auto GenerateBitmapFromSVGPath(const std::string& svg_path,
SDFMode mode,
double range,
Math::
Vec2i& center, double scale = 1, bool square = false) -> BitmapRGB - Generates a BitmapRGB from a SVG path using msdfgen's signed distance field method.
Enum documentation
enum OrbitEngine:: Graphics:: SimulationSpace
#include <OE/Graphics/2D/ParticleEmitter.hpp>
Enumerators | |
---|---|
LOCAL |
Particles will stay relative to the emitter. |
WORLD |
Particles positions will decouple from the emitter once created. |
enum OrbitEngine:: Graphics:: PathRendererCommandType
#include <OE/Graphics/2D/PathRenderer.hpp>
enum OrbitEngine:: Graphics:: MaterialMapType
#include <OE/Graphics/3D/Material.hpp>
enum OrbitEngine:: Graphics:: BufferType
#include <OE/Graphics/API/Buffer.hpp>
enum OrbitEngine:: Graphics:: BufferMode
#include <OE/Graphics/API/Buffer.hpp>
enum OrbitEngine:: Graphics:: BlitOperation
#include <OE/Graphics/API/FrameBuffer.hpp>
enum OrbitEngine:: Graphics:: MappedPointerMode
#include <OE/Graphics/API/MappedResource.hpp>
enum OrbitEngine:: Graphics:: Topology
#include <OE/Graphics/API/Mesh.hpp>
enum OrbitEngine:: Graphics:: ShaderType
#include <OE/Graphics/API/Shader.hpp>
enum OrbitEngine:: Graphics:: ShaderUniformType
enum OrbitEngine:: Graphics:: BlendState
#include <OE/Graphics/API/States.hpp>
enum OrbitEngine:: Graphics:: CullMode
#include <OE/Graphics/API/States.hpp>
enum OrbitEngine:: Graphics:: FunctionMode
#include <OE/Graphics/API/States.hpp>
enum OrbitEngine:: Graphics:: StencilOperation
#include <OE/Graphics/API/States.hpp>
enum OrbitEngine:: Graphics:: TextureFormat
#include <OE/Graphics/API/Texture.hpp>
enum OrbitEngine:: Graphics:: TextureFilter
#include <OE/Graphics/API/Texture.hpp>
enum OrbitEngine:: Graphics:: TextureWrap
#include <OE/Graphics/API/Texture.hpp>
enum OrbitEngine:: Graphics:: TextureDataType
#include <OE/Graphics/API/Texture.hpp>
enum OrbitEngine:: Graphics:: TextureDimension
#include <OE/Graphics/API/Texture.hpp>
enum OrbitEngine:: Graphics:: GlyphRenderMode
#include <OE/Graphics/Font.hpp>
Example: Grinning Face emoji (U+1F600) and **&** glyphs at 128px
GRAY
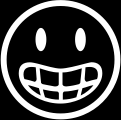

SDF/PSDF
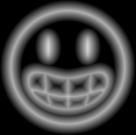
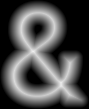
MSDF
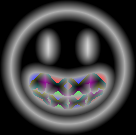
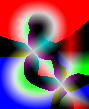
COLOR
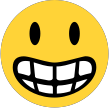

Enumerators | |
---|---|
GRAY |
monochrome |
SDF | |
PSDF | |
MSDF | |
COLOR |
full color with alpha (e.g. emojis) |
Typedef documentation
typedef std::map<ShaderType, std::string>OrbitEngine:: Graphics:: ShaderSources
#include <OE/Graphics/API/Shader.hpp>
typedef std::map<ShaderType, ShaderReflection>OrbitEngine:: Graphics:: ShaderReflections
#include <OE/Graphics/API/Shader.hpp>
typedef std::set<std::string>OrbitEngine:: Graphics:: ShaderDefinitions
#include <OE/Graphics/API/Shader.hpp>
typedef unsigned intOrbitEngine:: Graphics:: FrameIndex
#include <OE/Graphics/Atlas.hpp>
Frame identifier within an atlas. It must be unique and does not have to be sequential.
typedef Bitmap<unsigned char, 3>OrbitEngine:: Graphics:: BitmapRGB
#include <OE/Graphics/Bitmap.hpp>
typedef Bitmap<unsigned char, 4>OrbitEngine:: Graphics:: BitmapRGBA
#include <OE/Graphics/Bitmap.hpp>
typedef BitmapAtlas<unsigned char, 3>OrbitEngine:: Graphics:: BitmapAtlasRGB
#include <OE/Graphics/BitmapAtlas.hpp>
typedef BitmapAtlas<unsigned char, 4>OrbitEngine:: Graphics:: BitmapAtlasRGBA
#include <OE/Graphics/BitmapAtlas.hpp>
typedef unsigned intOrbitEngine:: Graphics:: GlyphCodepoint
#include <OE/Graphics/Font.hpp>
Unicode codepoint.
typedef unsigned intOrbitEngine:: Graphics:: FontSize
#include <OE/Graphics/Font.hpp>
Font sizes specified in pixels.
Function documentation
#include <OE/Graphics/Bitmap.hpp>
template<typename T, unsigned int N, typename U, unsigned int M>
Bitmap<U, M> OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<T, N>& input)
Convert between bitmaps.
Example: convert a 8bit grayscale image into RGB
Bitmap<unsigned char, 1> bitmap_grayscale(128, 128); // ... do something with bitmap_grayscale bitmap_grayscale.savePNG("image-8bit.png"); BitmapRGB bitmap_rgb = ConvertBitmap(bitmap_grayscale); // or Bitmap<unsigned char, 3> bitmap_rgb.savePNG("image-24bit.png");
#include <OE/Graphics/Bitmap.hpp>
template<>
BitmapRGB OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<unsigned char, 1>& input)
Convert from unsigned char 8bit (grayscale) to unsigned char 24bit (RGB)
#include <OE/Graphics/Bitmap.hpp>
template<>
BitmapRGBA OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<unsigned char, 1>& input)
Convert from unsigned char 8bit (grayscale) to unsigned char 32bit (RGBA)
#include <OE/Graphics/Bitmap.hpp>
template<>
BitmapRGBA OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<unsigned char, 3>& input)
Convert from unsigned char 24bit (RGB) to unsigned char 32bit (RGBA)
#include <OE/Graphics/Bitmap.hpp>
template<>
BitmapRGB OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<unsigned char, 4>& input)
Convert from unsigned char 32bit (RGBA) to unsigned char 24bit (RGB) (drop alpha)
#include <OE/Graphics/Bitmap.hpp>
template<>
BitmapRGB OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<float, 1>& input)
Convert from float 8bit (grayscale) to unsigned char 24bit (RGB)
#include <OE/Graphics/Bitmap.hpp>
template<>
BitmapRGB OrbitEngine:: Graphics:: ConvertBitmap(const Bitmap<float, 3>& input)
Convert from float 24bit (RGB) to unsigned char 24bit (RGB)
BitmapRGBA OrbitEngine:: Graphics:: ConvertBGRAtoRGBA(const Bitmap<unsigned char, 4>& input)
#include <OE/Graphics/Bitmap.hpp>
Convert a RGBA bitmap from BGRA to RGBA.
BitmapRGBA OrbitEngine:: Graphics:: ConvertAlphaToWhiteRGBA(const Bitmap<unsigned char, 1>& input)
#include <OE/Graphics/Bitmap.hpp>
unsigned char* OrbitEngine:: Graphics:: ReadImage(const std::string& path,
unsigned int& width,
unsigned int& height,
unsigned int& bpp,
bool force32bits = false)
#include <OE/Graphics/FreeImage.hpp>
Reads an image from disk.
Parameters | |
---|---|
path in | File location |
width out | Read image dimensions |
height out | Read image dimensions |
bpp out | Read image bits per pixel |
force32bits in | Force any 24bit image to load as a 32bit image |
Returns | Raw pointer to the pixel data. Remember to delete it after its used |
unsigned char* OrbitEngine:: Graphics:: ReadImage(unsigned char* file_data,
size_t file_size,
unsigned int& width,
unsigned int& height,
unsigned int& bpp,
bool force32bits = false)
#include <OE/Graphics/FreeImage.hpp>
Reads an image from disk.
Parameters | |
---|---|
file_data in | file binary data |
file_size in | file size |
width out | Read image dimensions |
height out | Read image dimensions |
bpp out | Read image bits per pixel |
force32bits in | Force any 24bit image to load as a 32bit image |
Returns | Raw pointer to the pixel data. Remember to delete it after its used |
bool OrbitEngine:: Graphics:: WritePNG(const std::string& path,
unsigned int width,
unsigned int height,
unsigned int bpp,
unsigned char* data)
#include <OE/Graphics/FreeImage.hpp>
Writes a PNG file to disk.
Parameters | |
---|---|
path in | File location |
width in | Image dimensions |
height in | Image dimensions |
bpp in | Bits per pixel present int the image |
data in | Raw pointer to the pixel data. Make sure it has at least width * height * bpp bits |
Returns | Whether the image was saved successfully |
BitmapRGB OrbitEngine:: Graphics:: GenerateBitmapFromOutline(FT_ Outline* outline,
SDFMode mode,
double range)
#include <OE/Graphics/MSDF.hpp>
Generates a BitmapRGB from a FreeType outline using msdfgen's signed distance field method.
Parameters | |
---|---|
outline in | FreeType's outline |
mode in | See SDFMode. |
range in | Width of the range around the shape between the minimum and maximum representable signed distance in pixels |
Returns | The generated bitmap, that can be invalid if the generation failed |
BitmapRGB OrbitEngine:: Graphics:: GenerateBitmapFromSVGPath(const std::string& svg_path,
SDFMode mode,
double range,
Math:: Vec2i& center,
double scale = 1,
bool square = false)
#include <OE/Graphics/MSDF.hpp>
Generates a BitmapRGB from a SVG path using msdfgen's signed distance field method.
Parameters | |
---|---|
svg_path in | SVG path (e.j "M150 0 L75 200 L225 200 Z") |
mode in | See SDFMode. |
range in | Width of the range around the shape between the minimum and maximum representable signed distance in pixels |
center out | corrected origin position after translation |
scale in | scale all coordinates by this factor |
square in | force a 1:1 w/h ratio |
Returns | The generated bitmap, that can be invalid if the generation failed |