class
#include <OE/Graphics/FontCollection.hpp>
FontCollection A collection of Glyphs and metadata.
Contents
Example: create a collection of text and emojis (no SDF)
const FontSize size = 22; Font* font = new Font("latin.ttf"); Font* font_emojis = new Font("emojis.ttf"); // create an empty collection FontCollection* collection = new FontCollection(); // add all the glyphs available in the text font collection->addGlyphs(font, font->getAvailableGlyphs(), size, GlyphRenderMode::COLOR); // filter the emoji font to only keep true emojis auto emojis = font_emojis->getAvailableGlyphs(); emojis.erase(std::remove_if(emojis.begin(), emojis.end(), [](const GlyphCodepoint& code) { return !Font::HasEmojiPresentation(code); }), emojis.end()); // add the glyphs to the collection collection->addGlyphs(emojis_font, emojis, size, GlyphRenderMode::COLOR); // export collection->exportToFiles("font-collection-fnt.json", "font-collection.json", "font-collection.png"); // cleanup delete collection;
The resulting font-collection.png:
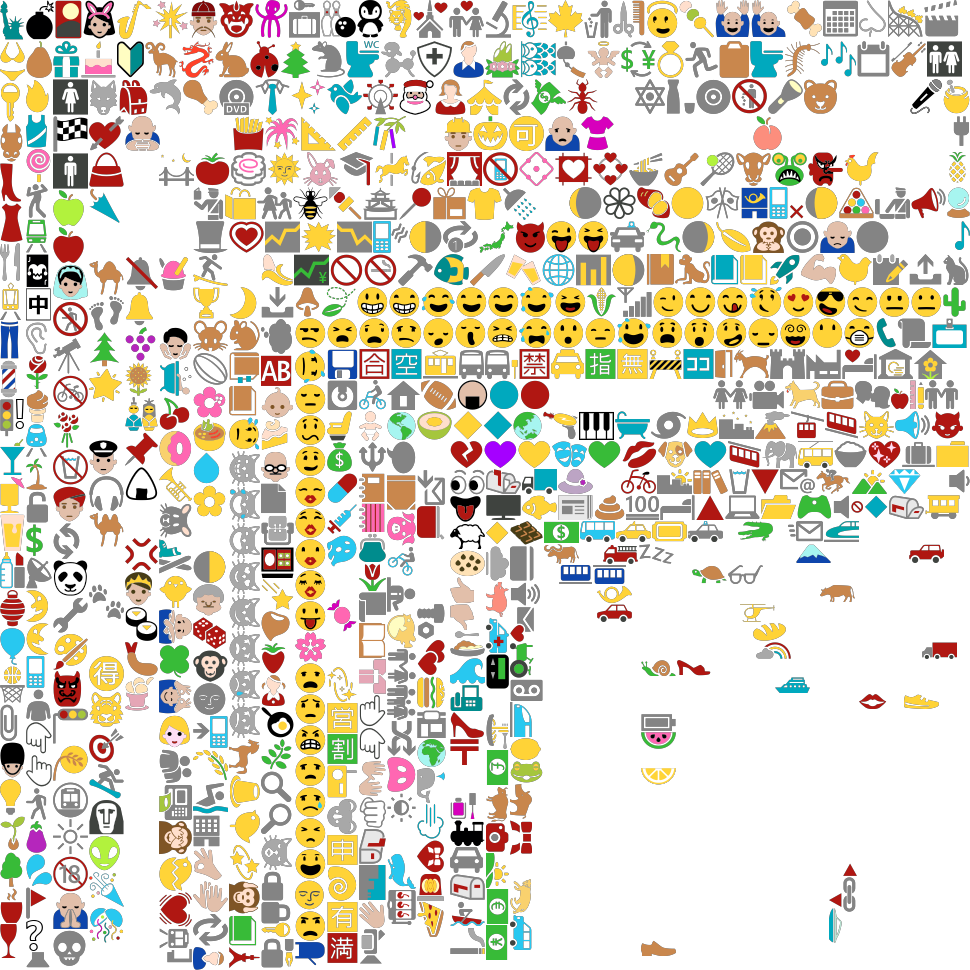
Metadata format:
{ "glyphs": [ { "i": 87, // codepoint "m": 4, // render mode "s": 22, // original font size "w": 19, // width "h": 14, // height "ha": 19, // horizontal advance "va": 19, // vertical advance "hbx": 0, // horizontal bearing X "hby": 14, // horizontal bearing Y "vbx": -10, // vertical bearing X "vby":2, // vertical bearing Y "k": [ // kernings, can be missing { "i": 228, // right codepoint "d": -1 // kerning distance }, ... ] }, ... ] }
Public static functions
- static auto Load(const std::string& font_metadata, const std::string& atlas_metadata, const std::string& atlas_image) -> FontCollection*
- Loads a font collection from disk.
- static auto toIndex(GlyphCodepoint code, GlyphRenderMode mode) -> FrameIndex
Constructors, destructors, conversion operators
- FontCollection()
- Creates an empty collection.
- ~FontCollection()
Public functions
- auto addGlyph(Font* font, GlyphCodepoint codepoint, FontSize size, GlyphRenderMode mode) -> bool
- Add a single glyph to the collection.
- auto addGlyphs(Font* font, const std::vector<GlyphCodepoint>& codepoints, FontSize size, GlyphRenderMode mode) -> int
- Add a group of glyph to the collection.
- auto exportToFiles(const std::string& font_metadata, const std::string& atlas_metadata, const std::string& atlas_image) -> bool
- Export the font collection to disk.
Function documentation
static FontCollection* OrbitEngine::Graphics::FontCollection:: Load(const std::string& font_metadata,
const std::string& atlas_metadata,
const std::string& atlas_image)
Loads a font collection from disk.
Parameters | |
---|---|
font_metadata in | path to the font metadata file to read (.json) |
atlas_metadata in | path to the atlas metadata fke to read (.json) |
atlas_image in | path to the atlas image file to read (.png) |
Returns | The FontCollection instance if the operation was successful, NULL otherwise |
bool OrbitEngine::Graphics::FontCollection:: addGlyph(Font* font,
GlyphCodepoint codepoint,
FontSize size,
GlyphRenderMode mode)
Add a single glyph to the collection.
Parameters | |
---|---|
font in | Font to extract the glyph |
codepoint in | glyph unicode's codepoint |
size in | target size to generate the glyph |
mode in | mode used to render the glyph |
Returns | Whether the glyph has been generated and added successfully |
int OrbitEngine::Graphics::FontCollection:: addGlyphs(Font* font,
const std::vector<GlyphCodepoint>& codepoints,
FontSize size,
GlyphRenderMode mode)
Add a group of glyph to the collection.
Parameters | |
---|---|
font in | Font to extract the glyphs |
codepoints in | list of the glyphs code points to add |
size in | target size to generate the glyphs |
mode in | mode used to render the glyphs |
Returns | The number of glyphs that have been generated and added successfully |
bool OrbitEngine::Graphics::FontCollection:: exportToFiles(const std::string& font_metadata,
const std::string& atlas_metadata,
const std::string& atlas_image)
Export the font collection to disk.
Parameters | |
---|---|
font_metadata in | path to write the font metadata file (.json) |
atlas_metadata in | path to write the atlas metadata file (.json) |
atlas_image in | path to write the atlas image file (.png) |
Returns | Whether the collection was exported correctly |